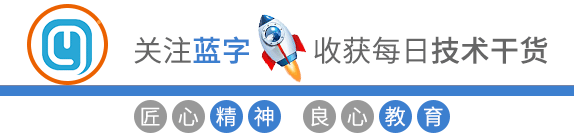
字符串操作
1.字符串的翻转
# 方式一
s = 'hello world'
print(s[::-1)
# 方式二
from functools import reduce
print(reduce(lambda x,y:y+x, s))
2.判断字符串是否是回文
利用字符串翻转操作可以查看字符串是否回文
s1 = 'abccba'
s2 = 'abcde'
def func(s):
if s == s[::-1]:
print(‘回文’)
else:
print('不回文')
func(s1)
func(s2)
3.寻找字符串中唯一的元素
去重操作可以借助 set 来进行
# 字符串
s1 = 'wwweeerftttg'
print(''.join(set(s1))) # ftgwer
# 列表
l1 = [2, 4, 5, 6, 7, 1, 2]
print(list(set(l1))) # [1, 2, 4, 5, 6, 7]
4.判断字符串所含元素是否相同
判断字符串中包含的元素是否相同,无论字符串中元素顺序如何,只要包含相同的元素和数量,就认为其是相同的。
from collections import Counter
s1, s2, s3 = 'asdf', 'fdsa', 'sfad'
c1, c2, c3 = Counter(s1), Counter(s2), Counter(s3)
if c1 == c2 and c2 == c3:
print('符合')
列表操作
1.将嵌套列表展开
from iteration_utilities import deepflatten
#Python小白学习交流群:153708845
l = [[12, 5, 3], [2. 4, [5], [6, 9, 7]], ]
print(list(deepflatten(l)))
2.从任意长度的可迭代对象中分解元素
first, *middle, last = grades #*表达式可以用来将一个含有N个元素的数据结构类型分解成所需的几部分
3.找到最大或最小的N个元素
import heapq
nums = [1, 8, 2, 23, 7, -4, 18, 23, 42, 37, 2]
print(heapq.nlargest(3, nums)) # [42, 37, 23]
print(heapq.nsmallest(3,nums)) # [-4, 1, 2]
# 根据指定的键得到最小的3个元素
portfolio = [
{'name': 'IBM', 'shares': 100, 'price': 91.1},
{'name': 'AAPL', 'shares': 50, 'price': 543.22},
{'name': 'FB', 'shares': 200, 'price': 21.09},
{'name': 'HPQ', 'shares': 35, 'price': 31.75},
{'name': 'YHOO', 'shares': 45, 'price': 16.35},
{'name': 'ACME', 'shares': 75, 'price': 115.65}
]
cheap = heapq.nsmallest(3, portfolio, key=lambda s: s['price'])
其他
1.检查对象的内存占用情况
import sys
s1 = 'a'
s2 = 'aaddf'
n1 = 32
print(sys.getsizeof(s1)) # 50
print(sys.getsizeof(s2)) # 54
print(sys.getsizeof(n1)) # 28
2.print操作
# print输出到文件
with open('somefile.txt', 'rt') as f:
print('Hello World!', file=f)
f.close()
# 以不同的分隔符或行结尾符完成打印
print('GKY',1995,5,18, sep='-',end='!!\n') # GKY-1995-5-18!!
3.读写压缩的文件
import gzip
with open('somefile.gz', 'rt') as f:
text = f.read()
f.close()
#Python小白学习交流群:153708845
import bz2
with open('somefile.bz2', 'rt') as f:
text = f.read()
f.close()
import gzip
with open('somefile.gz', 'wt') as f:
f.write(text)
f.close()
import bz2
with open('somefile.bz', 'wt') as f:
f.write(text)
f.close()
链接:https://www.cnblogs.com/djdjdj123/p/17817777.html
(版权归原作者所有,侵删)